If you’ve looked at Keras models on Github, you’ve probably noticed that there are some different ways to create models in Keras. There’s the Sequential model which allows you to define an entire model in a single line, usually with some line breaks for readability, then there’s the functional interface that allows for more complicated model architectures, and there’s also the Model subclass which helps reusability. In this article, we’re going to explore the different ways to create models in Keras, along with their advantages and drawbacks to equip you with the knowledge you need to create your own machine learning models in Keras.
After you completing this tutorial, you will learn:
Different ways that Keras offers to build models
How to use the Sequential class, functional interface, and subclassing keras.Model to build Keras models
When to use the different methods to create Keras models
Let’s get started!
Three Ways to Build Machine Learning Models in Keras Photo by Mike Szczepanski. Some rights reserved.
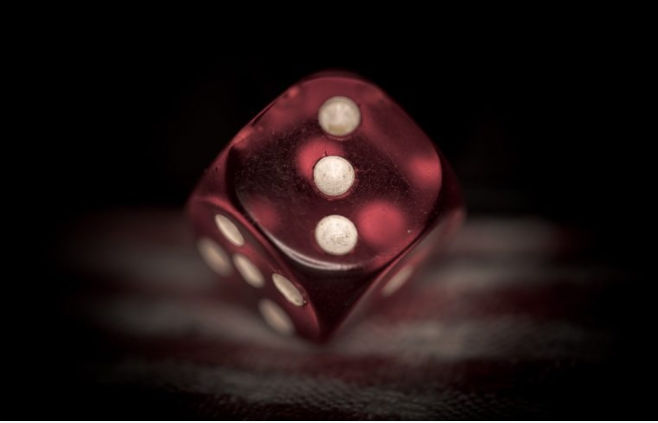
Overview
This tutorial is split into 3 parts, covering the different ways to building machine learning models in Keras:
Using the Sequential class
Using Keras’ functional interface
Subclassing keras.Model
Using the Sequential class
The Sequential Model is just as the name implies. It consists of a sequence of layers, one after the other. From the Keras documentation,
“A Sequential model is appropriate for a plain stack of layers where each layer has exactly one input tensor and one output tensor.”
It is a simple, easy-to-use way to get started building your Keras model. To start, import the Tensorflow, and then the Sequential model.
Using Keras’ Functional Interface
The next method of constructing Keras models that we’ll be exploring is using Keras’ functional interface. The functional interface uses the layers as functions instead, taking in a Tensor and outputting a Tensor as well. The functional interface is a more flexible way of representing a Keras model as we are not restricted only to sequential models that have layers stacked on top of one another. Instead, we can build models that branch into multiple paths, have multiple inputs, etc.
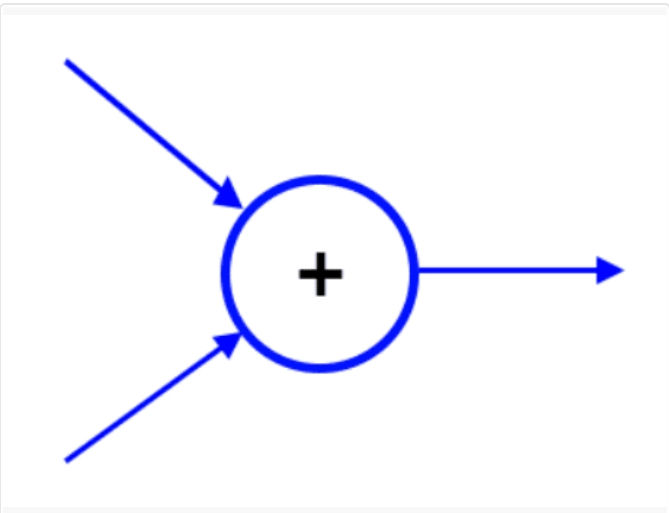
Further Reading
This section provides more resources on the topic if you are looking to go deeper.
Papers:
Deep Residual Learning for Image Recognition (the ResNet paper)
APIs:
Keras Sequential class
Keras functional API
Making new layers and models via subclassing